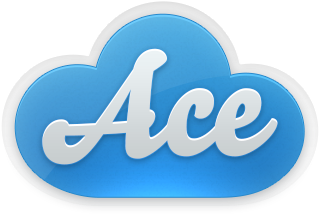
Built for Code
Ace is an embeddable code editor written in JavaScript. It matches the features and performance of native editors such as Sublime, Vim and TextMate. It can be easily embedded in any web page and JavaScript application. Ace is maintained as the primary editor for Cloud9 IDE and is the successor of the Mozilla Skywriter (Bespin) project.
/** * In fact, you're looking at ACE right now. Go ahead and play with it! * * We are currently showing off the JavaScript mode. ACE has support for 45 * language modes and 24 color themes! */ function add(x, y) { var resultString = "Hello, ACE! The result of your math is: "; var result = x + y; return resultString + result; } var addResult = add(3, 2); console.log(addResult);
Learn how to embed this in your own site
Looking for a more full-featured demo? Check out the kitchen sink.
Features
- Syntax highlighting for over 110 languages (TextMate/Sublime Text.tmlanguage files can be imported)
- Over 20 themes (TextMate/Sublime Text .tmtheme files can be imported)
- Automatic indent and outdent
- An optional command line
- Handles huge documents (four million lines seems to be the limit!)
- Fully customizable key bindings including vim and Emacs modes
- Search and replace with regular expressions
- Highlight matching parentheses
- Toggle between soft tabs and real tabs
- Displays hidden characters
- Drag and drop text using the mouse
- Line wrapping
- Code folding
- Multiple cursors and selections
- Live syntax checker (currently JavaScript/CoffeeScript/CSS/XQuery)
- Cut, copy, and paste functionality
Get the Open-Source Code
Ace is a community project. We actively encourage and support contributions! The Ace source code is hosted on GitHub and released under the BSD license ‐ very simple and friendly to all kinds of projects, whether open-source or not. Take charge of your editor and add your favorite language highlighting and keybindings!
git clone git://github.com/ajaxorg/ace.git
History
Skywriter/Bespin and Ace started as two independent projects both aiming to build a no compromise code editor component for the web. Bespin started as part of Mozilla Labs and was based on the <canvas> tag, while Ace is the editor component of Cloud9 IDE and uses the DOM for rendering. After the release of Ace at JSConf.eu 2010 in Berlin the Skywriter team decided to merge Ace with a simplified version of Skywriter's plugin system and some of Skywriter's extensibility points. All these changes have been merged back to Ace now, which supersedes Skywriter. Both Cloud9 IDE and Mozilla are actively developing and maintaining Ace.
Related Projects
Getting Help
Embedding Ace in Your Site
Ace can be easily embedded into a web page. Get prebuilt version of ace from ace-builds repository and use the code below:
<!DOCTYPE html> <html lang="en"> <head> <title>ACE in Action</title> <style type="text/css" media="screen"> #editor { position: absolute; top: 0; right: 0; bottom: 0; left: 0; } </style> </head> <body> <div id="editor">function foo(items) { var x = "All this is syntax highlighted"; return x; }</div> <script src="/ace-builds/src-noconflict/ace.js" type="text/javascript" charset="utf-8"></script> <script> var editor = ace.edit("editor"); editor.setTheme("ace/theme/monokai"); editor.session.setMode("ace/mode/javascript"); </script> </body> </html>
Now check out the How-To Guide for instructions on common operations, such as setting a different language mode or getting the contents from the editor.
Loading Ace from a Local URL
If you want to clone and host Ace locally you can
use one of the pre-packaged versions. Just copy
one of src*
subdirectories somewhere into your project, or use RequireJS to load the
contents of lib/ace folder as ace
:
Loading Ace from a CDN
The packaged version can also be loaded from CDN's such as jsDelivr or cdnjs.
Working with Ace
In all of these examples Ace has been invoked as shown in the embedding guide.
Configuring the editor
there are several ways to pass configuration to Ace
// pass options to ace.edit
ace.edit(element, {
mode: "ace/mode/javascript",
selectionStyle: "text"
})
// use setOptions method to set several options at once
editor.setOptions({
autoScrollEditorIntoView: true,
copyWithEmptySelection: true,
});
// use setOptions method
editor.setOption("mergeUndoDeltas", "always");
// some options are also available as methods e.g.
editor.setTheme(...)
// to get the value of the option use
editor.getOption("optionName");
See Configuring-Ace wiki page for a more detailed list of options.
Changing the size of the editor
Ace only checks for changes of the size of it's container when window is resized. If you resize the editor div in another manner, and need Ace to resize, use the following:
editor.resize()
if you want editor to change it's size based on contents, use maxLines option as shown in https://ace.c9.io/demo/autoresize.html
Setting Themes
Themes are loaded on demand; all you have to do is pass the string name:
editor.setTheme("ace/theme/twilight");
See all themes. Or use themelist extension to get the list of available themes at runtime.
Setting the Programming Language Mode
By default, the editor supports plain text mode. All other language modes are available as separate modules, loaded on demand like this:
editor.session.setMode("ace/mode/javascript");
One Editor, Multiple Sessions
Ace keeps everything about the state of the editor (selection, scroll position, etc.)
in editor.session
. This means you can grab the
session, store it in a var, and set the editor to another session (e.g. a tabbed editor).
You might accomplish this like so:
var js = ace.createEditSession("some js code");
var css = ace.createEditSession(["some", "css", "code here"]);
// and then to load document into editor, just call
editor.setSession(js);
Common Operations
Set and get content:
editor.setValue("the new text here");
editor.setValue("text2", -1); // set value and move cursor to the start of the text
editor.session.setValue("the new text here"); // set value and reset undo history
editor.getValue(); // or session.getValue
Get selected text:
editor.getSelectedText(); // or for a specific range
editor.session.getTextRange(editor.getSelectionRange());
Insert at cursor, emulating user input:
editor.insert("Something cool");
Replace text in range:
editor.session.replace(new ace.Range(0, 0, 1, 1), "new text");
Get the current cursor line and column:
editor.selection.getCursor();
Go to a line:
editor.gotoLine(lineNumber);
Get total number of lines:
editor.session.getLength();
Set the default tab size:
editor.session.setTabSize(4);
Use soft tabs:
editor.session.setUseSoftTabs(true);
Set the font size:
document.getElementById('editor').style.fontSize='12px';
Toggle word wrapping:
editor.session.setUseWrapMode(true);
Set line highlighting:
editor.setHighlightActiveLine(false);
Set the print margin visibility:
editor.setShowPrintMargin(false);
Set the editor to read-only:
editor.setReadOnly(true); // false to make it editable
Using undo manager
To group undo delta of the next edit with the previous one set `mergeUndoDeltas` to true
editor.session.mergeUndoDeltas = true;
editor.session.insert({row: 0, column:0}, Date()+"");
To start new undo group use `markUndoGroup` method
editor.insertSnippet("a$0b");
editor.session.markUndoGroup();
editor.insertSnippet("x$0y");
To disable undo of a an edit in a collaborative editor
var rev = session.$undoManager.startNewGroup(); // start new undo group
... // apply the edit
session.$undoManager.markIgnored(rev); // mark the new group as ignored
To implement undo/redo buttons see https://ace.c9.io/demo/toolbar.html
Searching
editor.find('needle',{
backwards: false,
wrap: false,
caseSensitive: false,
wholeWord: false,
regExp: false
});
editor.findNext();
editor.findPrevious();
The following options are available to you for your search parameters:
-
needle
: The string or regular expression you're looking for -
backwards
: Whether to search backwards from where cursor currently is. Defaults tofalse
. -
wrap
: Whether to wrap the search back to the beginning when it hits the end. Defaults tofalse
. -
caseSensitive
: Whether the search ought to be case-sensitive. Defaults tofalse
. -
wholeWord
: Whether the search matches only on whole words. Defaults tofalse
. -
range
: The Range to search within. Set this tonull
for the whole document -
regExp
: Whether the search is a regular expression or not. Defaults tofalse
. -
start
: The starting Range or cursor position to begin the search -
skipCurrent
: Whether or not to include the current line in the search. Default tofalse
. -
preventScroll
: Whether or not to move the cursor to the next match. Default tofalse
.
Here's how you can perform a replace:
editor.find('foo');
editor.replace('bar');
And here's a replace all:
editor.replaceAll('bar');
(editor.replaceAll
uses the needle set earlier by editor.find('needle', ...
)
Listening to Events
To listen for an onchange
:
editor.session.on('change', function(delta) {
// delta.start, delta.end, delta.lines, delta.action
});
To listen for an selection
change:
editor.session.selection.on('changeSelection', function(e) {
});
To listen for a cursor
change:
editor.session.selection.on('changeCursor', function(e) {
});
Adding New Commands and Keybindings
To assign key bindings to a custom function:
editor.commands.addCommand({
name: 'myCommand',
bindKey: {win: 'Ctrl-M', mac: 'Command-M'},
exec: function(editor) {
//...
},
readOnly: true, // false if this command should not apply in readOnly mode
// multiSelectAction: "forEach", optional way to control behavior with multiple cursors
// scrollIntoView: "cursor", control how cursor is scolled into view after the command
});
Configure dynamic loading of modes and themes
By default ace detcts the url for dynamic loading by finding the script node for ace.js. This doesn't work if ace.js is not loaded with a separate script tag, and in this case it is required to set url explicitely
ace.config.set("basePath", "https://url.to.a/folder/that/contains-ace-modes");
Path for one module alone can be configured with:
ace.config.setModuleUrl("ace/theme/textmate", "url for textmate.js");
When using ace with webpack, it is possible to configure paths for all submodules using
require("ace-builds/esm-resolver"); // for new bundlers: webpack 5, rollup, vite
For webpack 4 use
require("ace-builds/webpack-resolver");
which depends on file-loader
Creating a Syntax Highlighter for Ace
Creating a new syntax highlighter for Ace is extremely simple. You'll need to define two pieces of code: a new mode, and a new set of highlighting rules.
Where to Start
We recommend using the Ace Mode Creator when defining your highlighter. This allows you to inspect your code's tokens, as well as providing a live preview of the syntax highlighter in action.
Defining a Mode
Every language needs a mode. A mode contains the paths to a language's syntax highlighting rules, indentation rules, and code folding rules. Without defining a mode, Ace won't know anything about the finer aspects of your language.
Here is the starter template we'll use to create a new mode:
define(function(require, exports, module) {
"use strict";
var oop = require("../lib/oop");
// defines the parent mode
var TextMode = require("./text").Mode;
var Tokenizer = require("../tokenizer").Tokenizer;
var MatchingBraceOutdent = require("./matching_brace_outdent").MatchingBraceOutdent;
// defines the language specific highlighters and folding rules
var MyNewHighlightRules = require("./mynew_highlight_rules").MyNewHighlightRules;
var MyNewFoldMode = require("./folding/mynew").MyNewFoldMode;
var Mode = function() {
// set everything up
this.HighlightRules = MyNewHighlightRules;
this.$outdent = new MatchingBraceOutdent();
this.foldingRules = new MyNewFoldMode();
};
oop.inherits(Mode, TextMode);
(function() {
// configure comment start/end characters
this.lineCommentStart = "//";
this.blockComment = {start: "/*", end: "*/"};
// special logic for indent/outdent.
// By default ace keeps indentation of previous line
this.getNextLineIndent = function(state, line, tab) {
var indent = this.$getIndent(line);
return indent;
};
this.checkOutdent = function(state, line, input) {
return this.$outdent.checkOutdent(line, input);
};
this.autoOutdent = function(state, doc, row) {
this.$outdent.autoOutdent(doc, row);
};
// create worker for live syntax checking
this.createWorker = function(session) {
var worker = new WorkerClient(["ace"], "ace/mode/mynew_worker", "NewWorker");
worker.attachToDocument(session.getDocument());
worker.on("errors", function(e) {
session.setAnnotations(e.data);
});
return worker;
};
}).call(Mode.prototype);
exports.Mode = Mode;
});
What's going on here? First, you're defining the path to TextMode
(more on this later). Then you're pointing the mode to your definitions for the highlighting rules, as well as your rules for code folding. Finally, you're setting everything up to find those rules, and exporting the Mode so that it can be consumed. That's it!
Regarding TextMode
, you'll notice that it's only being used once: oop.inherits(Mode, TextMode);
. If your new language depends on the rules of another language, you can choose to inherit the same rules, while expanding on it with your language's own requirements. For example, PHP inherits from HTML, since it can be embedded directly inside .html pages. You can either inherit from TextMode
, or any other existing mode, if it already relates to your language.
All Ace modes can be found in the lib/ace/mode folder.
Defining Syntax Highlighting Rules
The Ace highlighter can be considered to be a state machine. Regular expressions define the tokens for the current state, as well as the transitions into another state. Let's define mynew_highlight_rules.js, which our mode above uses.
All syntax highlighters start off looking something like this:
define(function(require, exports, module) {
"use strict";
var oop = require("../lib/oop");
var TextHighlightRules = require("./text_highlight_rules").TextHighlightRules;
var MyNewHighlightRules = function() {
// regexp must not have capturing parentheses. Use (?:) instead.
// regexps are ordered -> the first match is used
this.$rules = {
"start" : [
{
token: token, // String, Array, or Function: the CSS token to apply
regex: regex, // String or RegExp: the regexp to match
next: next // [Optional] String: next state to enter
}
]
};
};
oop.inherits(MyNewHighlightRules, TextHighlightRules);
exports.MyNewHighlightRules = MyNewHighlightRules;
});
The token state machine operates on whatever is defined in this.$rules
. The highlighter always begins at the start
state, and progresses down the list, looking for a matching regex
. When one is found, the resulting text is wrapped within a <span class="ace_<token>">
tag, where <token>
is defined as the token
property. Note that all tokens are preceded by the ace_
prefix when they're rendered on the page.
Once again, we're inheriting from TextHighlightRules
here. We could choose to make this any other language set we want, if our new language requires previously defined syntaxes. For more information on extending languages, see "extending Highlighters" below.
Defining Tokens
The Ace highlighting system is heavily inspired by the TextMate language grammar. Most tokens will follow the conventions of TextMate when naming grammars. A thorough (albeit incomplete) list of tokens can be found on the Ace Wiki.
For the complete list of tokens, see tool/tmtheme.js. It is possible to add new token names, but the scope of that knowledge is outside of this document.
Multiple tokens can be applied to the same text by adding dots in the token, e.g. token: support.function
wraps the text in a <span class="ace_support ace_function">
tag.
Defining Regular Expressions
Regular expressions can either be a RegExp or String definition
If you're using a regular expression, remember to start and end the line with the /
character, like this:
{
token : "constant.language.escape",
regex : /\$[\w\d]+/
}
A caveat of using stringed regular expressions is that any \
character must be escaped. That means that even an innocuous regular expression like this:
regex: "function\s*\(\w+\)"
Must actually be written like this:
regex: "function\\s*\(\\w+\)"
Groupings
You can also include flat regexps--(var)
--or have matching groups--((a+)(b+))
. There is a strict requirement whereby matching groups must cover the entire matched string; thus, (hel)lo
is invalid. If you want to create a non-matching group, simply start the group with the ?:
predicate; thus, (hel)(?:lo)
is okay. You can, of course, create longer non-matching groups. For example:
{
token : "constant.language.boolean",
regex : /(?:true|false)\b/
},
For flat regular expression matches, token
can be a String, or a Function that takes a single argument (the match) and returns a string token. For example, using a function might look like this:
var colors = lang.arrayToMap(
("aqua|black|blue|fuchsia|gray|green|lime|maroon|navy|olive|orange|" +
"purple|red|silver|teal|white|yellow").split("|")
);
var fonts = lang.arrayToMap(
("arial|century|comic|courier|garamond|georgia|helvetica|impact|lucida|" +
"symbol|system|tahoma|times|trebuchet|utopia|verdana|webdings|sans-serif|" +
"serif|monospace").split("|")
);
...
{
token: function(value) {
if (colors.hasOwnProperty(value.toLowerCase())) {
return "support.constant.color";
}
else if (fonts.hasOwnProperty(value.toLowerCase())) {
return "support.constant.fonts";
}
else {
return "text";
}
},
regex: "\\-?[a-zA-Z_][a-zA-Z0-9_\\-]*"
}
If token
is a function,it should take the same number of arguments as there are groups, and return an array of tokens.
For grouped regular expressions, token
can be a String, in which case all matched groups are given that same token, like this:
{
token: "identifier",
regex: "(\\w+\\s*:)(\\w*)"
}
More commonly, though, token
is an Array (of the same length as the number of groups), whereby matches are given the token of the same alignment as in the match. For a complicated regular expression, like defining a function, that might look something like this:
{
token : ["storage.type", "text", "entity.name.function"],
regex : "(function)(\\s+)([a-zA-Z_][a-zA-Z0-9_]*\\b)"
}
Defining States
The syntax highlighting state machine stays in the start
state, until you define a next
state for it to advance to. At that point, the tokenizer stays in that new state
, until it advances to another state. Afterwards, you should return to the original start
state.
Here's an example:
this.$rules = {
"start" : [ {
token : "text",
regex : "<\\!\\[CDATA\\[",
next : "cdata"
} ],
"cdata" : [ {
token : "text",
regex : "\\]\\]>",
next : "start"
}, {
defaultToken : "text"
} ]
};
In this extremely short sample, we're defining some highlighting rules for when Ace detects a <![CDATA
tag. When one is encountered, the tokenizer moves from start
into the cdata
state. It remains there, applying the text
token to any string it encounters. Finally, when it hits a closing ]>
symbol, it returns to the start
state and continues to tokenize anything else.
Using the TMLanguage Tool
There is a tool that will take an existing tmlanguage file and do its best to convert it into Javascript for Ace to consume. Here's what you need to get started:
- In the Ace repository, navigate to the tools folder.
- Run
npm install
to install required dependencies. - Run
node tmlanguage.js <path_to_tmlanguage_file>
; for example,node <path_to_tmlanguage_file> /Users/Elrond/elven.tmLanguage
Two files are created and placed in lib/ace/mode: one for the language mode, and one for the set of highlight rules. You will still need to add the code into ace/ext/modelist.js, and add a sample file for testing.
A Note on Accuracy
Your .tmlanguage file will then be converted to the best of the converter’s ability. It is an understatement to say that the tool is imperfect. Probably, language mode creation will never be able to be fully autogenerated. There's a list of non-determinable items; for example:
- The use of regular expression lookbehinds
This is a concept that JavaScript simply does not have and needs to be faked - Deciding which state to transition to
While the tool does create new states correctly, it labels them with generic terms likestate_2
,state_10
, e.t.c. - Extending modes
Many modes say something likeinclude source.c
, to mean, “add all the rules in C highlighting.” That syntax does not make sense to Ace or this tool (though of course you can extending existing highlighters). - Rule preference order
- Gathering keywords
Most likely, you’ll need to take keywords from your language file and run them throughcreateKeywordMapper()
However, the tool is an excellent way to get a quick start, if you already possess a tmlanguage file for you language.
Extending Highlighters
Suppose you're working on a LuaPage, PHP embedded in HTML, or a Django template. You'll need to create a syntax highlighter that takes all the rules from the original language (Lua, PHP, or Python) and extends it with some additional identifiers (<?lua
, <?php
, {%
, for example). Ace allows you to easily extend a highlighter using a few helper functions.
Getting Existing Rules
To get the existing syntax highlighting rules for a particular language, use the getRules()
function. For example:
var HtmlHighlightRules = require("./html_highlight_rules").HtmlHighlightRules;
this.$rules = new HtmlHighlightRules().getRules();
/*
this.$rules == Same this.$rules as HTML highlighting
*/
Extending a Highlighter
The addRules
method does one thing, and it does one thing well: it adds new rules to an existing rule set, and prefixes any state with a given tag. For example, let's say you've got two sets of rules, defined like this:
this.$rules = {
"start": [ /* ... */ ]
};
var newRules = {
"start": [ /* ... */ ]
}
If you want to incorporate newRules
into this.$rules
, you'd do something like this:
this.addRules(newRules, "new-");
/*
this.$rules = {
"start": [ ... ],
"new-start": [ ... ]
};
*/
Extending Two Highlighters
The last function available to you combines both of these concepts, and it's called embedRules
. It takes three parameters:
- An existing rule set to embed with
- A prefix to apply for each state in the existing rule set
- A set of new states to add
Like addRules
, embedRules
adds on to the existing this.$rules
object.
To explain this visually, let's take a look at the syntax highlighter for Lua pages, which combines all of these concepts:
var HtmlHighlightRules = require("./html_highlight_rules").HtmlHighlightRules;
var LuaHighlightRules = require("./lua_highlight_rules").LuaHighlightRules;
var LuaPageHighlightRules = function() {
this.$rules = new HtmlHighlightRules().getRules();
for (var i in this.$rules) {
this.$rules[i].unshift({
token: "keyword",
regex: "<\\%\\=?",
next: "lua-start"
}, {
token: "keyword",
regex: "<\\?lua\\=?",
next: "lua-start"
});
}
this.embedRules(LuaHighlightRules, "lua-", [
{
token: "keyword",
regex: "\\%>",
next: "start"
},
{
token: "keyword",
regex: "\\?>",
next: "start"
}
]);
};
Here, this.$rules
starts off as a set of HTML highlighting rules. To this set, we add two new checks for <%=
and <?lua=
. We also delegate that if one of these rules are matched, we should move onto the lua-start
state. Next, embedRules
takes the already existing set of LuaHighlightRules
and applies the lua-
prefix to each state there. Finally, it adds two new checks for %>
and ?>
, allowing the state machine to return to start
.
Code Folding
Adding new folding rules to your mode can be a little tricky. First, insert the following lines of code into your mode definition:
var MyFoldMode = require("./folding/newrules").FoldMode;
...
var MyMode = function() {
...
this.foldingRules = new MyFoldMode();
};
You'll be defining your code folding rules into the lib/ace/mode/folding folder. Here's a template that you can use to get started:
define(function(require, exports, module) {
"use strict";
var oop = require("../../lib/oop");
var Range = require("../../range").Range;
var BaseFoldMode = require("./fold_mode").FoldMode;
var FoldMode = exports.FoldMode = function() {};
oop.inherits(FoldMode, BaseFoldMode);
(function() {
// regular expressions that identify starting and stopping points
this.foldingStartMarker;
this.foldingStopMarker;
this.getFoldWidgetRange = function(session, foldStyle, row) {
var line = session.getLine(row);
// test each line, and return a range of segments to collapse
};
}).call(FoldMode.prototype);
});
Just like with TextMode
for syntax highlighting, BaseFoldMode
contains the starting point for code folding logic. foldingStartMarker
defines your opening folding point, while foldingStopMarker
defines the stopping point. For example, for a C-style folding system, these values might look like this:
this.foldingStartMarker = /(\{|\[)[^\}\]]*$|^\s*(\/\*)/;
this.foldingStopMarker = /^[^\[\{]*(\}|\])|^[\s\*]*(\*\/)/;
These regular expressions identify various symbols--{
, [
, //
--to pay attention to. getFoldWidgetRange
matches on these regular expressions, and when found, returns the range of relevant folding points. For more information on the Range
object, see the Ace API documentation.
Again, for a C-style folding mechanism, a range to return for the starting fold might look like this:
var line = session.getLine(row);
var match = line.match(this.foldingStartMarker);
if (match) {
var i = match.index;
if (match[1])
return this.openingBracketBlock(session, match[1], row, i);
var range = session.getCommentFoldRange(row, i + match[0].length);
range.end.column -= 2;
return range;
}
Let's say we stumble across the code block hello_world() {
. Our range object here becomes:
{
startRow: 0,
endRow: 0,
startColumn: 0,
endColumn: 13
}
Testing Your Highlighter
The best way to test your tokenizer is to see it live, right? To do that, you'll want to modify the live Ace demo to preview your changes. You can find this file in the root Ace directory with the name kitchen-sink.html.
-
add an entry to
supportedModes
inace/ext/modelist.js
-
add a sample file to
demo/kitchen-sink/docs/
with same name as the mode file
Once you set this up, you should be able to witness a live demonstration of your new highlighter.
Adding Automated Tests
Adding automated tests for a highlighter is trivial so you are not required to do it, but it can help during development.
In lib/ace/mode/_test
create a file named
with some example code. (You can skip this if the document you have added in text_<modeName>.txt
demo/docs
both looks good and covers various edge cases in your language syntax).
Run node highlight_rules_test.js -gen
to preserve current output of your tokenizer in tokens_<modeName>.json
After this running highlight_rules_test.js optionalLanguageName
will compare output of your tokenizer with the correct output you've created.
Any files ending with the _test.js suffix are automatically run by Ace's Travis CI server.
Ace API Reference
Welcome to the Ace API Reference!
On the left, you'll find a list of all of our currently documented classes. These is not a complete set of classes, but rather, the "core" set. For more information on how to work with Ace, check out the embedding guide.
Below is an ERD diagram describing some fundamentals about how the internals of Ace works:
Projects Using Ace
Ace is used all over the web in all kinds of production applications. Here is just a small sampling: